We all remember those "How to learn C++ in 21 days" books:
Many people are probably skeptical about this idea, but (as we’ve already been into the future) there is no reason to doubt it? Before we delve into the specifics of our programming venture, here is a brief introduction to our team. We are a couple of programmers with no claim (oh, really?) to fame like Angry Birds and, moreover, with very little experience in iOS or GameDev in general. Roughly speaking, for us, this project is being undertaken as both a challenge to ourselves and, ultimately, for the pure fun and experience even if little can be achieved (oh, really?) in the end.
Now that you know at least a little more about us than Google can tell you, let’s talk about the rules for this endeavor. “Two weeks are enough for every project” was a statement made by one of us (let’s call him Bill…No, Bond is better) in a Skype call late one night. “Okay, but maybe…” was the reply that was abruptly cut off by “No buts or maybes.” Well, OK, if you say so! Of course, we could spend these weeks investing time in our families, working, taking care of the fate of humanity, or any number of other noble pursuits. Yeah, right! If you’ll buy that, I have a bridge to sell you. If we weren’t doing this project, the honest truth is that we’d be playing Starcraft, wasting time on Facebook, or just drinking beer.
Anyway, back to the rules, which have been set:
Two weeks of deeply intensive development is enough if you think about it. After all, 2 * 7 * 24 = 336 hours, which translates, via the following sophisticated math (336/8 *7/5 = 58.8), into nearly 59 calendar days or 2 whole months! Clearly the math justifies the time limit (looks like there might be a trick somewhere… But millions of managers can be wrong!). And, quite seriously (this is a serious post; honestly), our programming practices prove that the best way to raise the priority of any given task is to decrease the time limits for completing it. So, exactly two weeks (“exactly” means two weeks plus an extra two weeks depending on the situation). Rule 1 is complete!
Work on other rules later.
OK, now that the time limit is set, we must decide what we should write. After an objective evaluation of our capabilities (who are we trying to fool?), we decide not to write a 4D-shooter with RPG (or BDSM) elements and multiplayer capabilities for 20,000,000 simultaneous players. However, after some discussion, we do think that having a simpler multiplayer option our game is a grand idea.
As a basis, we took the game called GIPF, as there is nothing in the AppStore (and not planned, as we see). We decided to change the rules of the original game a little bit to make it even simpler to play, to ensure that it is not an exact copy of the original game, and to make it even more fun. In short, it will be a logical game with rules a bit more complicated than Tic-tac-toe and with a bit less need for strategy than chess. This seems like a good way for roughly 67 to 68% of Americans to spend their time. This number of users is calculated using extremely strict formulas with basic expectations about the number of sales of the application, at the price of $149, that are needed in order for us to purchase an island in the Mediterranean Sea on which to live and carry on about our programming. These numbers can be slightly reduced (~1,000 fold) in case we decide that money is not the main goal of our lives.
Our plans for the day:
High-level architectural design of the application
List of tasks and time limits
Make decisions about the supported platforms and iOS version
Day 2.
Today there won't be any jokes because the serious development process has started. Let's begin on a positive note: we still have some development time left and our time milestone hasn’t passed yet. While being serious, we should look at what our current decisions are:
We will use Cocos2d as the graphical engine (cocos2d for iPhone)
The minimum target iOS version will be 4.2 or 5.0 (there are some good reasons). This decision has been made after analysis of the iOS statistics for August 2011 (http://www.marco.org/2011/08/13/instapap...ts-update)
Initial architecture thoughts:
Model: low level
We thought that when it comes to AI and processing tons of data, any overhead would be multiplied by a large factor (maybe several thousand fold). As a result, we decided to write the low-level (everything that is connected with data representation and the board state) in pure C.
The two base classes are:
* BoardInfo – This contains data that does not change during the whole game: board parameters: width/height, field structure, etc.
* BoardState – This contains data that changes during the game: current set of pieces on board, number of pieces in reserve for both players, etc.
Our AI thoughts are based on evaluation of a large quantity of board states (for example, if we use Alpha-Beta pruning), so we absolutely need to use simple 'memcpy' to copy them and the fastest operations to operate with them. That's why the data organization above and pure C were chosen.
Model: high level
Here comes Objective-C and high-level wrappers of the two classes discussed above. AI uses low-level model only to make decisions about what is the “best move.” In all other cases, high level model objects are used (even to make the move, which was just evaluated using low-level structures only).
HexBoardGame – This class contains inner variables of type BoardInfo that do not change during the game. It also contains an inner variable of type BoardState, which is changed every turn, as well as initialization functions. Everything that is needed to create a game skeleton from board parameters (BoardInfo) and changing board states (BoardState) is also included in this class.
GipfBoardGame – This contains the specific implementation of HexBoardGame for iGipf rules. It expands HexBoardGame with such things as moves with pieces shifting (see the rules), row removal (see the rules), etc.
Controller
Here, we think everything is clear.
Views
Everything is even clearer here. CCScene -- is a class from Cocos2d.
Conclusion:
Enough for today. These drafts helped us to understand what we are going to implement and how to start the basic coding routine. The next interesting step will be AI experiments for which we need a proof-of-concept; but we'll talk about that.
_________________________________________________
Edited by Moderator - blog liks are not allowed, please read the forum rules
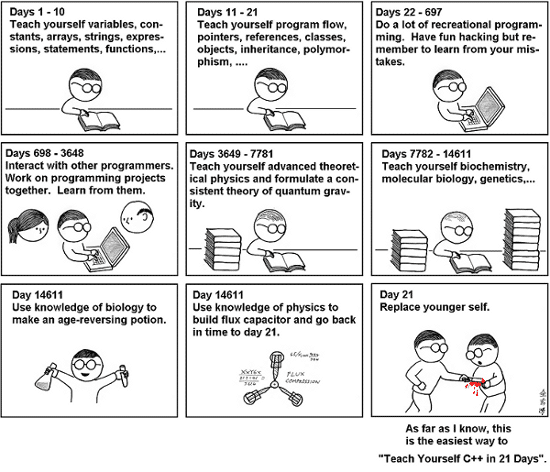
Many people are probably skeptical about this idea, but (as we’ve already been into the future) there is no reason to doubt it? Before we delve into the specifics of our programming venture, here is a brief introduction to our team. We are a couple of programmers with no claim (oh, really?) to fame like Angry Birds and, moreover, with very little experience in iOS or GameDev in general. Roughly speaking, for us, this project is being undertaken as both a challenge to ourselves and, ultimately, for the pure fun and experience even if little can be achieved (oh, really?) in the end.
Now that you know at least a little more about us than Google can tell you, let’s talk about the rules for this endeavor. “Two weeks are enough for every project” was a statement made by one of us (let’s call him Bill…No, Bond is better) in a Skype call late one night. “Okay, but maybe…” was the reply that was abruptly cut off by “No buts or maybes.” Well, OK, if you say so! Of course, we could spend these weeks investing time in our families, working, taking care of the fate of humanity, or any number of other noble pursuits. Yeah, right! If you’ll buy that, I have a bridge to sell you. If we weren’t doing this project, the honest truth is that we’d be playing Starcraft, wasting time on Facebook, or just drinking beer.
Anyway, back to the rules, which have been set:
Two weeks of deeply intensive development is enough if you think about it. After all, 2 * 7 * 24 = 336 hours, which translates, via the following sophisticated math (336/8 *7/5 = 58.8), into nearly 59 calendar days or 2 whole months! Clearly the math justifies the time limit (looks like there might be a trick somewhere… But millions of managers can be wrong!). And, quite seriously (this is a serious post; honestly), our programming practices prove that the best way to raise the priority of any given task is to decrease the time limits for completing it. So, exactly two weeks (“exactly” means two weeks plus an extra two weeks depending on the situation). Rule 1 is complete!
Work on other rules later.
OK, now that the time limit is set, we must decide what we should write. After an objective evaluation of our capabilities (who are we trying to fool?), we decide not to write a 4D-shooter with RPG (or BDSM) elements and multiplayer capabilities for 20,000,000 simultaneous players. However, after some discussion, we do think that having a simpler multiplayer option our game is a grand idea.
As a basis, we took the game called GIPF, as there is nothing in the AppStore (and not planned, as we see). We decided to change the rules of the original game a little bit to make it even simpler to play, to ensure that it is not an exact copy of the original game, and to make it even more fun. In short, it will be a logical game with rules a bit more complicated than Tic-tac-toe and with a bit less need for strategy than chess. This seems like a good way for roughly 67 to 68% of Americans to spend their time. This number of users is calculated using extremely strict formulas with basic expectations about the number of sales of the application, at the price of $149, that are needed in order for us to purchase an island in the Mediterranean Sea on which to live and carry on about our programming. These numbers can be slightly reduced (~1,000 fold) in case we decide that money is not the main goal of our lives.
Our plans for the day:
High-level architectural design of the application
List of tasks and time limits
Make decisions about the supported platforms and iOS version
Day 2.
Today there won't be any jokes because the serious development process has started. Let's begin on a positive note: we still have some development time left and our time milestone hasn’t passed yet. While being serious, we should look at what our current decisions are:
We will use Cocos2d as the graphical engine (cocos2d for iPhone)
The minimum target iOS version will be 4.2 or 5.0 (there are some good reasons). This decision has been made after analysis of the iOS statistics for August 2011 (http://www.marco.org/2011/08/13/instapap...ts-update)
Initial architecture thoughts:

Model: low level
We thought that when it comes to AI and processing tons of data, any overhead would be multiplied by a large factor (maybe several thousand fold). As a result, we decided to write the low-level (everything that is connected with data representation and the board state) in pure C.
The two base classes are:
* BoardInfo – This contains data that does not change during the whole game: board parameters: width/height, field structure, etc.
* BoardState – This contains data that changes during the game: current set of pieces on board, number of pieces in reserve for both players, etc.
Our AI thoughts are based on evaluation of a large quantity of board states (for example, if we use Alpha-Beta pruning), so we absolutely need to use simple 'memcpy' to copy them and the fastest operations to operate with them. That's why the data organization above and pure C were chosen.
Model: high level
Here comes Objective-C and high-level wrappers of the two classes discussed above. AI uses low-level model only to make decisions about what is the “best move.” In all other cases, high level model objects are used (even to make the move, which was just evaluated using low-level structures only).
HexBoardGame – This class contains inner variables of type BoardInfo that do not change during the game. It also contains an inner variable of type BoardState, which is changed every turn, as well as initialization functions. Everything that is needed to create a game skeleton from board parameters (BoardInfo) and changing board states (BoardState) is also included in this class.
GipfBoardGame – This contains the specific implementation of HexBoardGame for iGipf rules. It expands HexBoardGame with such things as moves with pieces shifting (see the rules), row removal (see the rules), etc.
Controller
Here, we think everything is clear.
Views
Everything is even clearer here. CCScene -- is a class from Cocos2d.
Conclusion:
Enough for today. These drafts helped us to understand what we are going to implement and how to start the basic coding routine. The next interesting step will be AI experiments for which we need a proof-of-concept; but we'll talk about that.
_________________________________________________
Edited by Moderator - blog liks are not allowed, please read the forum rules
Last edited by a moderator: